Flushing Promises in JavaScript - what it is and why you need it in UI tests
“This UI test is flaky, why does it sometimes fail?” - “Could it be because promises were not flushed?” - “Huuuuh?” Chances are high that frontend developers will have had conversations like this. From my experience, many developers are still unaware of what flushing promises means and why we need it in automated tests of UI components such as with React or Angular. Let’s shed some light on this.
Why do I need to flush promises?
Consider the following case: I created a new UI component. On starting, it displays the text “Empty”. When the component renders, it starts a network request to a server. The server takes 1 second to return a response. Once the component receives the response, it replaces the text “Empty” with the response it received.
Expand to see a demo GIF of the component:
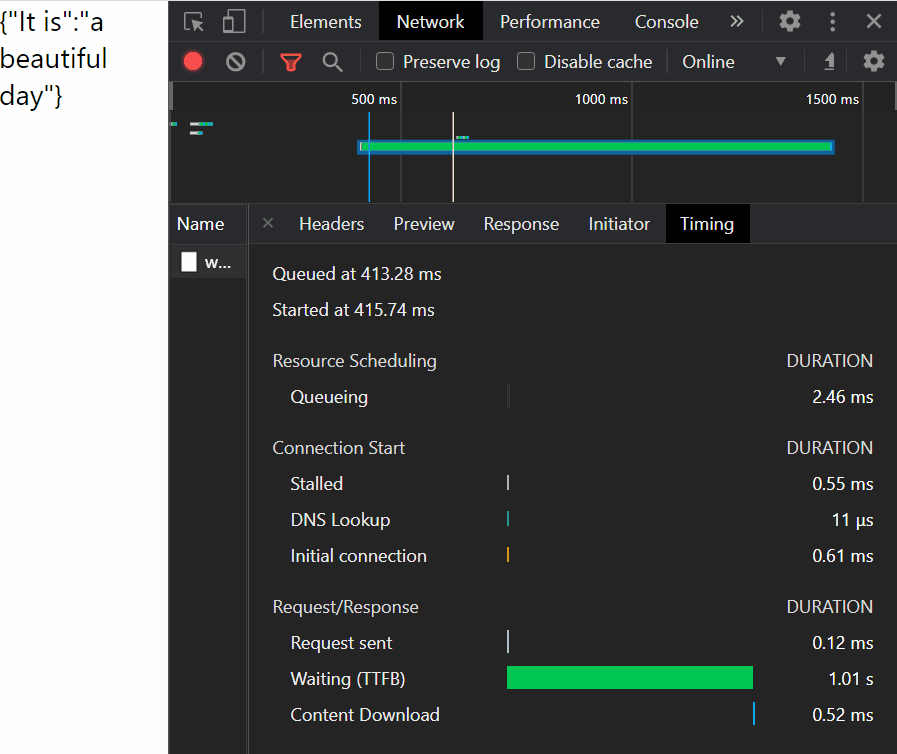
A demo of our dummy component
The code of this dummy component:
import React from "react"
export async function makeNetworkRequest() {
const response = await fetch("http://localhost:3001/what-day-is-it")
const data = await response.json()
return JSON.stringify(data)
}
class App extends React.Component {
constructor(props) {
super(props)
this.state = {
webResponse: "Empty",
}
}
async componentDidMount() {
const webResponse = await makeNetworkRequest()
this.setState({webResponse})
}
render() {
return (
<div className="App">
<div className="response">{this.state.webResponse}</div>
</div>
)
}
}
export default App
This component works, fantastic! So let’s write some automated tests for this.
First, let’s test our network request function in isolation. We will use the npm module nock to mock the network request that would normally be made. This allows us to run the tests without our server. It will essentially fake the network request and have it always return a fake response, the JSON string {"A": "mocked response"}
:
import App, {makeNetworkRequest} from "./App"
import nock from "nock"
const mockedWebResponse = JSON.stringify('{"A": "mocked response"}')
function setUpMock() {
nock(/.*/)
.persist()
.defaultReplyHeaders({
"access-control-allow-origin": "*",
"access-control-allow-credentials": "true",
})
.get(/what-day-is-it/)
.reply(200, mockedWebResponse)
}
it("Testing our network request", async () => {
setUpMock()
const result = await makeNetworkRequest()
expect(result).toEqual(mockedWebResponse)
})
This test passes, amazing. Take note that makeNetworkRequest
is an asynchronous function. Since we are using the async
and await
keywords, nodejs will hold up with the subsequent I/O executions and only populate the result
variable with a value once makeNetworkRequest
finishes.
Let’s move on to testing our component. We will render the component using the Testing Library npm module and then check whether the text shows our fixed response:
import {render} from "@testing-library/react"
it("Test if component renders web response", () => {
const component = render(<App />)
const response = component.container.querySelector(".response")
expect(response.textContent).toEqual(mockedWebResponse)
})
But this test is failing. Why? Because no promises have been flushed. Alternatively, because nodejs exited too early. What jest does here is executing the function inside the it
block which includes I/O operations only. But the update of the component where the text changes from “Empty” to our mocked response is not synchronous; this update happens in fact asynchronously because of our network request but also because of how React works internally. But since the test is not using any await
keyword, nodejs exits as soon as possible. And this is the major problem with UI tests: Components are observables which don’t return promises or values. Therefore, we cannot add await
keywords anywhere at will.
And this is why we need to flush promises. Let us first speak about how we could get the test to pass and we will then discuss why it works.
We can flush promises with the following function:
function flushPromises() {
return new Promise(setImmediate)
}
Since it returns a promise, it needs to be called using await
:
it("Test if component renders web response", async () => {
const component = render(<App />)
const response = component.container.querySelector(".response")
for (let index = 0; index < 200; index++) {
await flushPromises()
}
expect(response.textContent).toEqual(mockedWebResponse)
})
And there you go, the test passes now! Alternatively, we could also use the waitFor
helper exposed by the Testing Library. A deep-dive into the source code shows that it is essentially just a wrapper around our current solution which also uses setImmediate
- but the waitFor
helper doesn’t flush for a random 200 times but only as often as actually needed. This speeds up our test slightly.
import {render, waitFor} from "@testing-library/react"
it("Test if component renders web response", async () => {
const component = render(<App />)
const response = component.container.querySelector(".response")
await waitFor(() => expect(response.textContent).toEqual(mockedWebResponse))
})
How does flushing promises work
As you saw above, both solutions involved the function setImmediate
. This function is special. In fact, the MDN Web Docs mention that it should not be used in production websites. But since we only deal here with nodejs and tests, this doesn’t matter to us.
In order to know more about how setImmediate
works, we need to learn what the JavaScript event loop is. I will try my best to sum it up and paraphrase: JavaScript is a non-blocking programming language. This means that if I would make 10 network requests each taking 1 second then my program would not take 10 seconds but still only 1 second. This is achieved via the event loop which is essentially a task queue. JavaScript then takes the tasks from the task queue and executes them concurrently.
Imagine you are in school and your teacher gives you homework - you will not complete all of these tasks right the instance the teacher asked you to do so; instead, you will write down these tasks in your notebook and you will execute these tasks once it’s a convenient time to do so.
Sticking to the homework example, let’s pretend your teacher gave you 100 tasks of homework. But there is only so much you can do in one day, you only have time for 10 exercises per day. This is your “call stack size”. The nodejs event loop looks at all scheduled tasks and tries to put as many as possible onto the call stack so that they can be executed.
But here is where the function setImmediate
comes in. If the teacher would have given 100 tasks for the next week, nodejs would try to prioritise the tasks which the teacher gave the longest time ago in order to catch up. But functions which are scheduled using setImmediate
are special - these functions are additional tasks. This means that if your teacher gave you 100 tasks and 5 tasks scheduled using setImmediate
, nodejs will first do the 10 tasks it can do per day and then additionally also do all of the tasks queued up using setImmediate
. In essence, setImmediate
functions are extra tasks which take higher priority.
How does this translate onto our flushPromises
function? The above way of writing was implicit, so let’s pick it apart and write it explicitly:
function flushPromises() {
return new Promise((resolve, reject) => {
setImmediate(() => {
resolve()
})
})
}
What happens is that an asynchronous event (all functions returning promises are async functions) is being scheduled which contains a setImmediate
call. As we have learnt, setImmediate
is only called in the event loop after tasks from the call stack have been executed. Therefore the event loop is forced to execute tasks of the call stack first before it can call setImmediate. Our setImmediate
function is basically sandwiched into all of the other queued up tasks. Since we are returning a promise, we have again something to wait for using the await
keyword.
In essence, because setImmediate
always happens after the execution of tasks on the event loop, “flushing promises” is about forcing the event loop to execute some queued up tasks. But since the call stack is only limited (remember that we can only do 10 pieces of homework per day), we need to call it multiple times in order to force multiple cycles through the event loop.
And that’s flushing promises for you! I hope that this article both helped you understand the problem and also how to solve it.