mock-inspect: A simple way to achieve contract testing
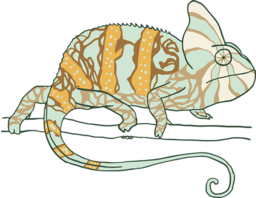
In last week’s article, we discussed how we can use mock-inspect to assert if and how the application under test makes network requests to API endpoints while simultaneously mocking out their responses. This week we are going to explore how we can use mock-inspect to implement a contract testing strategy without complicated frameworks like PACT.
This post is accompanied by a GitHub repository which holds all the code examples so that they can be tried out locally.
Understanding contract testing
A network request is made out of two steps:
- The consumer asks for something
- The provider answers with something
The problem is usually that provider and consumer sit in different code bases. Think of a web app: The provider would be the backend server and the consumer would be a frontend application questioning the backend server. Both projects usually live in separate repositories.
Contract testing aims to ensure that if the consumer asks for the right thing, the provider answers with the right thing. A popular testing framework for this is PACT which is a monster on its own, providing beefy implementations for a multitude of programming languages. The way it works is by setting up a “contract” which contains information about how the request should be made and what the API should respond with. To check the consumer side, PACT would tell you to create a mock server that responds with some mocked data given that the request has been made the right way.
mock-inspect is a Node.js tool which allows you to mock network requests and inspect how these requests were made. You can see where I am going with this: As long as the consumer is written in Node.js (like a frontend application or a backend server), we can use mock-inspect for contract testing - no need for the complicated PACT framework.
Strategy overview (no contracts yet)
In contract testing, we want to test both aspects of the flow: the provider and the consumer side.
A provider test is simple to do and doesn’t even need mock-inspect. As long as we write a test for our backend server which asserts that the response is correct (given the correct request was made), we have already the provider side covered. Pretty easy.
More interesting is the consumer side which is where mock-inspect can be used. No matter if your consumer is a backend server or a frontend application, as long as it is written in Node.js and making a network request, mock-inspect can help you. All we have to do is to write a test with a mocked network request and then assert that the consumer has asked the right question, i.e. has included the correct headers and payload. This is how such a consumer test could look like in mock-inspect:
const {mockRequest} = require("mock-inspect")
// Inside your test
// Step 1: Set up the mock for the third party API so that your test
// does not hit the real API. Provide a fixed response body.
const thirdPartyMock = mockRequest({
url: "http://localhost:5000/v1/cities_you_can_fly_to",
method: "POST",
responseBody: {
London: true,
Birmingham: true,
Edinburgh: false
}
})
// Step 2: Have your test execute the code which is expected
// to call the provider (backend server)
await printPossibleLocations()
// Step 3: Using mock-inspect's `inspect()` method available on
// instances returned by `mockRequest`, check out how the request
// was made and match it against your expectations
const {
requestPayload,
requestHeaders
} = thirdPartyMock.inspect()
expect(requestHeaders["authorization"]).toEqual("Bearer my_API_token_was_used")
expect(requestPayload).toEqual({filterByCountry: "England"})
The above test executes our function which is making a network request to our backend server (the “provider”) and then asserts that the request has been made correctly. In the example above, we are checking that the API token has been forwarded in the request headers and that a request payload has been used to filter the list of cities by a specific country.
Adding a contract
We now have two separate test suites which do their job. But the problem is that these tests don’t yet relate to each other: we could end up modifying the expectations on one side only to realise that these don’t match up anymore with the behaviour on the other side.
This is where contracts come in. A contract is a way of codifying the behaviour for both sides of the request, consumer and provider. The provider (the backend server) agrees to respond with a certain message as long as it is asked the correct question. The consumer in turn can be sure that it will receive the correct answer given it asks the right question.
A suitable format for this information is JSON as it can be understood by any programming language. That way, we can use contract testing even for non-Node.js providers, no matter if these are written in Java, Golang or something else. I would structure a contract as follows:
{
"request": {
"url": "http://localhost:5000/v1/cities_you_can_fly_to",
"method": "POST",
"payload": {
"type": "object",
"properties": {
"filterByCountry": {
"type": "string",
"minLength": 1
}
},
"additionalProperties": true,
"required": [
"filterByCountry"
]
},
"headers": {
"authorization": "Bearer my_API_token_was_used"
}
},
"response": {
"body": {
"type": "object",
"minProperties": 3,
"patternProperties": {
"^.*$": {
"type": "boolean"
}
}
},
"statusCode": 200,
"headers": {
"content-type": "application/json; charset=utf-8"
}
}
}
We are defining two pieces of information here:
- In
request
, we define how the request should be made. We should be hitting the desired URL with thePOST
method and we need to provide a JSON payload. The JSON payload needs to follow a specific JSON schema. To explain JSON schema would be out of scope for this article but in short, it is a way of describing JSON structures. In the case above, we say that thepayload
needs to be a JSON object with a required property calledfilterByCountry
which holds a string value. - In
response
, we define what the API should return. The API should respond with a status code of 200 and theapplication/json; charset=utf-8
header as Content-Type. The response body should be a JSON object with at least three properties which should all hold a boolean value.
These contracts are best placed into a separate repository so that they can be accessed by different test suites. Ideally, you would version this repository either using NPM modules or with git tags. Versioning allows for different test suites to use these contracts independently and makes updating test suites a breeze.
Once we have the contract finished, all we have to do is set up our two tests so that they make use of the contract instead of the previously hard-coded values. The provider test (for the backend server) will now take the JSON schema defined under response.body
and assert that the API does indeed serve a JSON structure matching that schema. The test will now also check that the status code and the headers match those defined in response
.
Below is how the provider test looks with contracts. We are using the NPM library json-schema-faker
to generate a sample request payload that abides by the rules stated in the contract’s JSON schema. Additionally, we are using the AJV library to assert that the response body matches the JSON schema.
const request = require("request-promise")
const contract = require("../../contracts/v1/cities_you_can_fly_to.json")
// Used to auto-generate a request payload from the contract's JSON schema
const jsonSchemaFaker = require("json-schema-faker")
// Used to validate that the response body matches the JSON schema
const AJV = require("ajv")
const ajv = new AJV({allErrors: true})
const result = await request({
url: contract.request.url,
method: contract.request.method,
headers: contract.request.headers,
body: jsonSchemaFaker.generate(contract.request.payload),
resolveWithFullResponse: true,
json: true,
})
expect(result.statusCode).toBe(contract.response.statusCode)
for (const header in contract.response.headers) {
expect(result.headers[header]).toBe(
contract.response.headers[header],
)
}
const validator = ajv.compile(contract.response.body)
const isValid = validator(result.body)
if (!isValid) {
throw new Error(
"Our provider did not send a response body matching" +
"the contract response body.",
)
}
The consumer test works similarly, but this time we are generating a sample response and validate against the request payload. Thanks to mock-inspect, we don’t need to run the backend server for our unit tests: mock-inspect mocks the outgoing network request and returns the specified sample response. We can make use of the inspect()
method exposed by mock-inspect to see how a request has been made. This is exactly what we want to assert on the consumer side: we want to ensure that the consumer makes the network request as stated in the contract.
This is how our consumer test looks like after introducing contracts:
const {printPossibleLocations} = require("../index.js")
const contract = require("../../contracts/v1/cities_you_can_fly_to.json")
// Used to mock the network request going to the API
const {mockRequest} = require("mock-inspect")
// Used to auto-generate a response from the contract's JSON schema
const jsonSchemaFaker = require("json-schema-faker")
// Used to validate that the request payload matches the JSON schema
const AJV = require("ajv")
const ajv = new AJV({allErrors: true})
const citiesRequest = mockRequest({
requestPattern: contract.request.url,
requestMethod: contract.request.method,
responseBody: jsonSchemaFaker.generate(contract.response.body),
responseStatus: contract.response.statusCode,
responseHeaders: contract.response.headers,
})
await printPossibleLocations()
const {requestBody, requestHeaders} = citiesRequest.inspect()
for (const header in contract.request.headers) {
expect(requestHeaders[header]).toBe(
contract.request.headers[header],
)
}
const validator = ajv.compile(contract.request.payload)
const isValid = validator(requestBody)
if (!isValid) {
throw new Error(
"Our consumer did not send a request payload matching" +
"the contract request payload.",
)
}
Closing words
As you saw, it is easy to do contract testing with mock-inspect, there is no need for complicated frameworks like PACT. All we need is a consumer written in Node.js so that we can use mock-inspect to mock network requests. Since any programming language can read JSON files, providers can make use of the contracts in their test suites, no matter if these provider services are written in Java, Golang or any other language - the same principles apply.
I wish you a lot of fun with contract testing! Should you have any questions, don’t hesitate to reach out to us over at mock-inspect.
Chameleon graphic by Анна Куликова from Pixabay